3D
For creating three dimensional sketches with p5, you will need to switch the default renderer (P2D) to WEBGL. In practice, this can be done by passing the constant WEBGL
to the createCanvas
function:
function setup() {
createCanvas(400, 400, WEBGL);
}
With the WEBGL renderer, there are six 3D primitives available in p5: plane
, box
, cylinder
, cone
, torus
and sphere
.
3D coordinate system
Note, that with the WEBGL renderer the coordinate 0,0 (x,y) is located at the center of the canvas, not in the top left corner as with the default renderer. In the 3D context there is also the third axis z, which is pointing towards you from the screen.
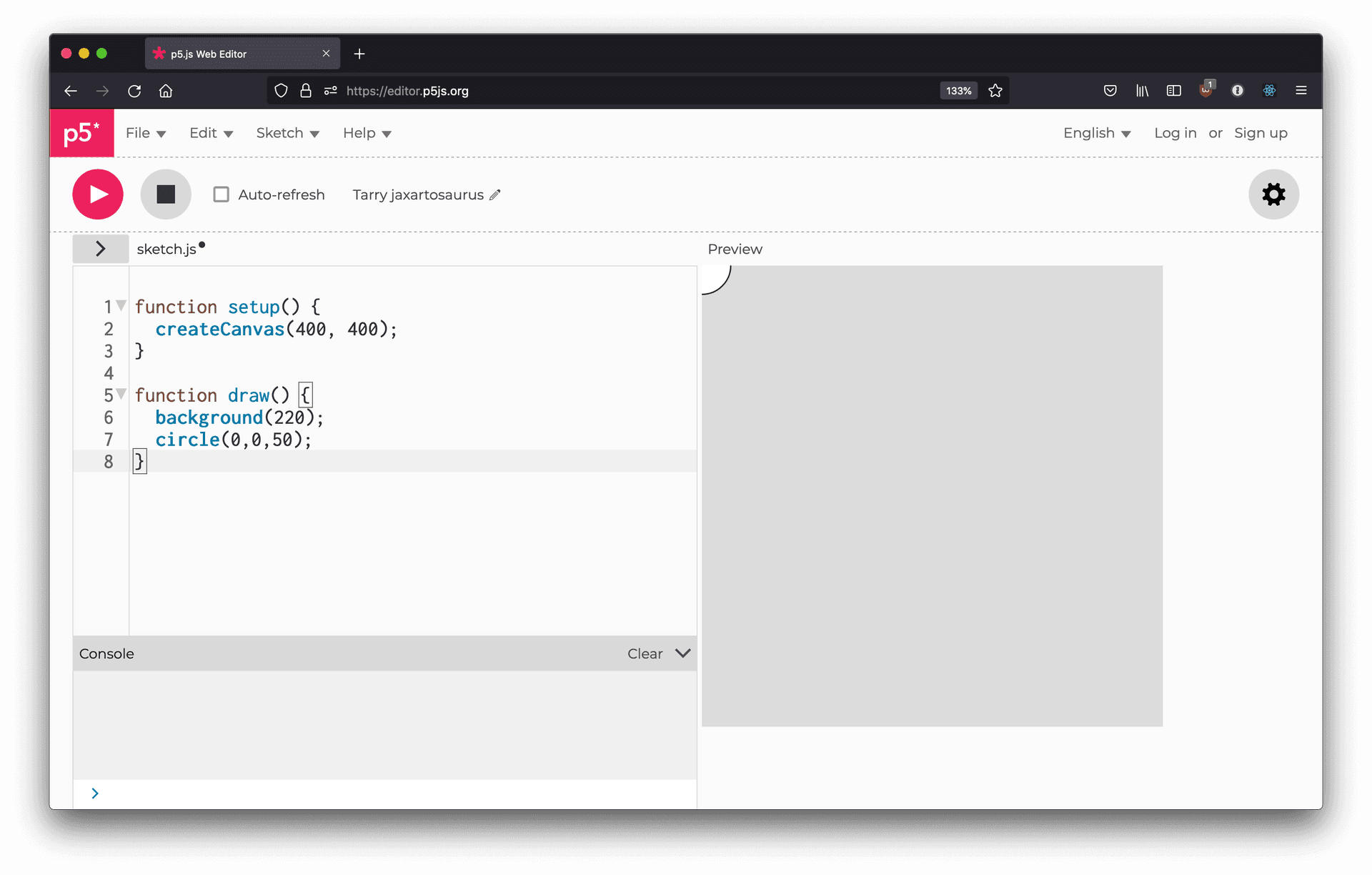
With the default renderer the point 0,0 (x,y) is located in the top left corner of the canvas.
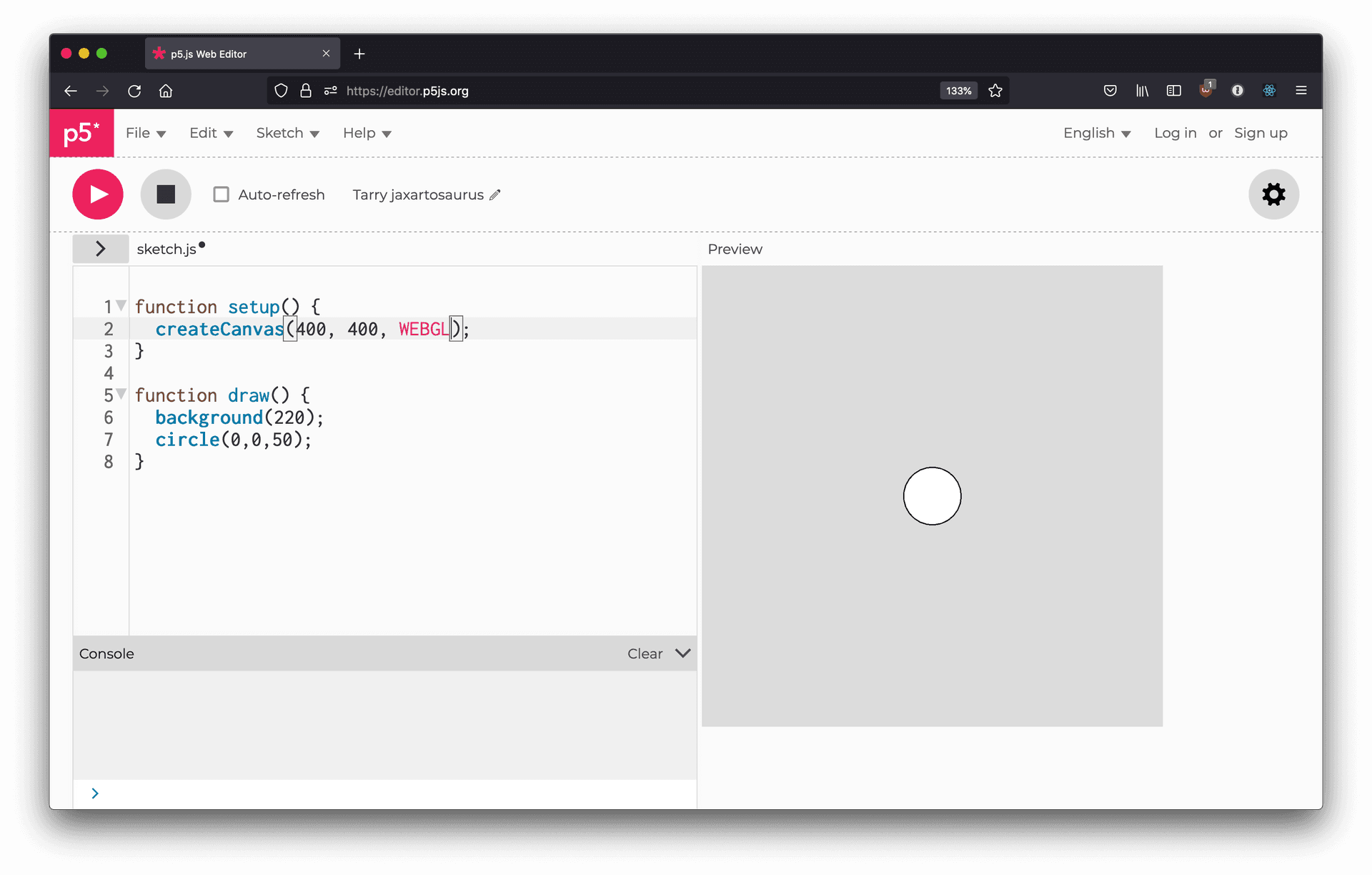
With the WEBGL renderer the point 0,0,0 (x,y,z) is located in the center of the canvas.
Positioning 3D primitives
Functions for the 3D primitives, such as box
do not take parameters for positioning. The 3D primitives will allways be drawn to 0,0,0 (x,y,z). In order to move the elements on the canvas, you need to call the translate function.
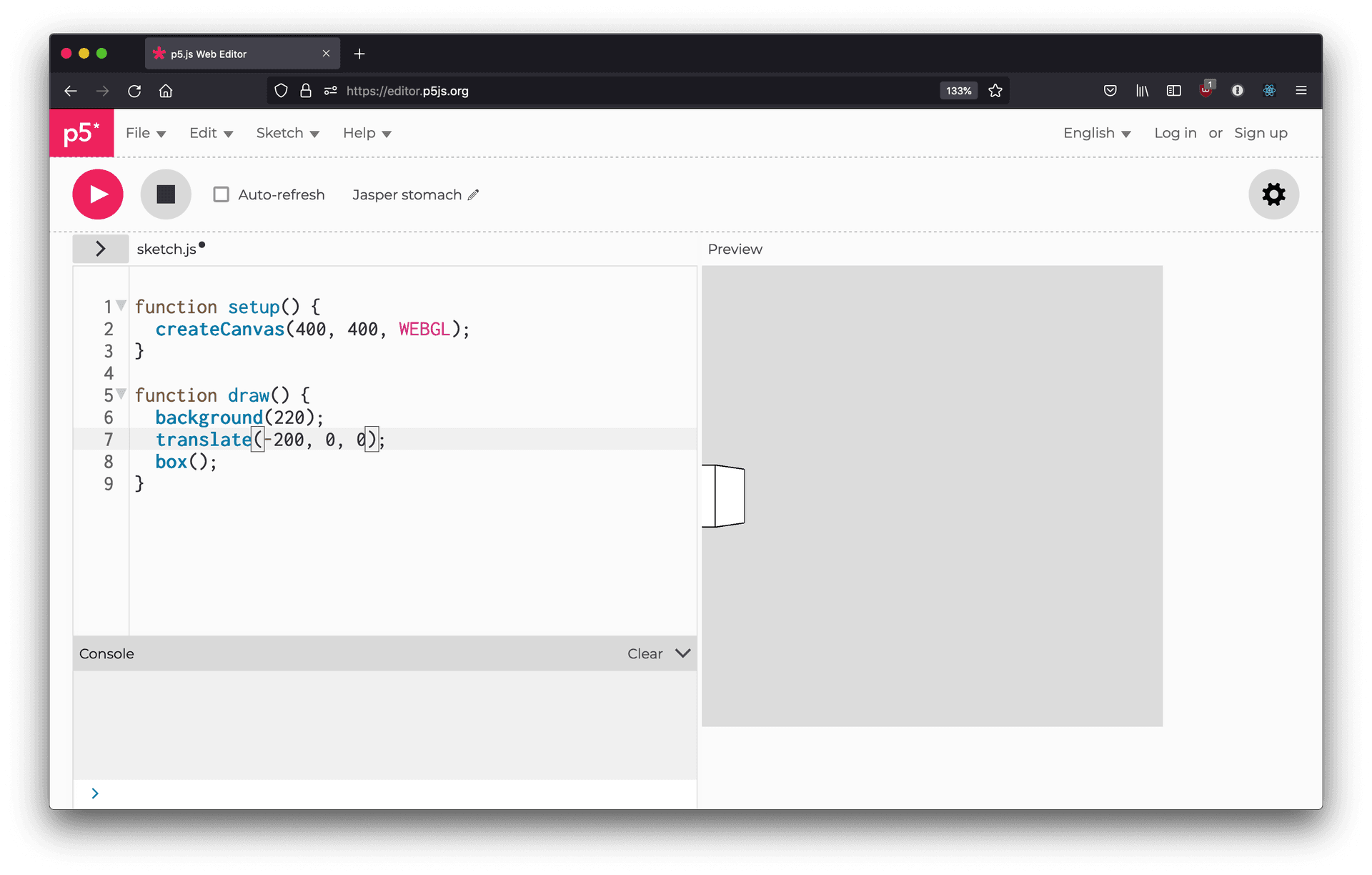
Positioning a box with the translate function.
3D Camera
The sketch is rendered through a virtual camera and it is possible to change different features of the camera with p5. You can for axample move the camera around and change the perspective. The available functions can be found from the reference under 'Camera'.
The default camera view in WEBGL mode is perspective with a 60 degree field of view. To override the default options, call perspective()
or ortho()
. In orthographic view (ortho()), objects of the same dimensions appear to be the same size even if they are farther away on the z-plane. The parameters for the ortho()
function define the bounding box with minimum and maximum values for the different axis. If no parameters are given, the following default is used: ortho(-width/2, width/2, -height/2, height/2).
Materials and light
You can use fill
and stroke
in the WEBGL context as you would in the default context. However, With WEBGL you can also use lights (ambientLight()
, directionalLight()
and pointLight()
) and materials (normalMaterial()
,
ambientMaterial()
and specularMaterial()
) for rendering. The materials work similarly to fill and stroke. Once you call a material function, that material is used untill another material or fill function is called.